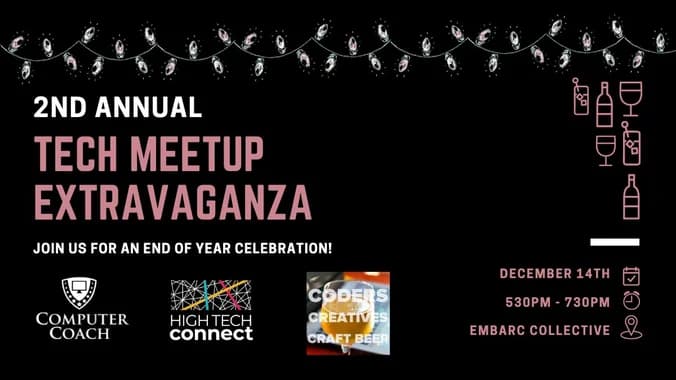
If you’re in Tampa Bay and in tech, a tech-adjacent field, or hoping to get into tech or a tech-adjacent field, you’ll want to attend the Annual Tech Meetup Extravaganza taking place at the Tampa Bay startup hub Embarc Collective on Wednesday, December 14th from 5:30 p.m. to 7:30 p.m.!
The event is FREE to attend — just RSVP on the event page!
Join me, Anitra, and a whole big swath of Tampa Bay’s tech scene at this “meetup of meetups,” where all of Tampa Bay’s tech meetups get together to celebrate the end of the year and the start of the holidays. As the organizers put it:
“2022 has been an exciting year for Tampa Bay and we’re grateful to be part of the growth and opportunity happening right here!”
“We’re bringing together tech meetup groups from across the bay for a night of celebration, networking, and the opportunity to decompress and reflect back on another year of hard work and accomplishments in the books!”
There’s be food provided by Glory Days Grill, drinks as well, and raffles — lots of raffles, according to the organizers. To participate in the raffles, bring an unwrapped gift of minimum $10 cash donation, which goes to the event’s toy drive.
Join us, along with these other Tampa Bay tech meetups (and more are getting added to this list daily!):
- Tampa Devs
- Tampa Bay Data Science Group
- Tampa Bay Tech Career Advice Forum
- Tech4Good Tampa
- Tampa Bay InfraGard
- Tampa Blacks in Technology Foundation
- Tampa Azure User Group
A big thank-you to:
- The event’s organizers, Computer Coach
- The co-sponsors, High Tech Connect
- The event hosts, Embarc Collective!
We’ll see you there!