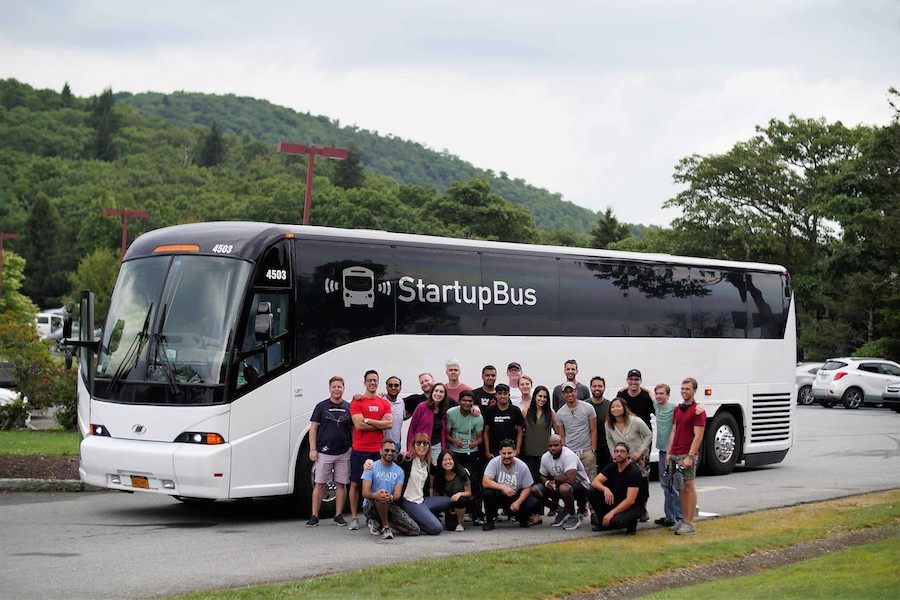
As the title of this post puts it: Everything you need to know in order to win StartupBus North America 2022 is contained within a podcast. This is the second in a series of posts covering the “Startup Bus” series of episodes from Gimlet Media’s Startup podcast, which covered the New York bus’ journey during StartupBus 2017.
If you haven’t yet seen the previous post, check it out! It covers episode 1 of the Startup Bus series, which introduces you to the buspreneurs on the 2017 New York bus.
Episode 2: It’s like one of those competition TV shows!
You can listen to episode 2 on its page or use the player below:
Here are my notes from this episode:
- Eric the narrator discovers the secret of StartupBus: it’s like a reality TV show, but in real life! “When I was growing up, my family was very into a particular kind of reality tv—competition shows… I thought I’d be reporting on a hackathon. I’d find one person, going through something interesting, and we’d just see how their week played out. Pretty simple. But when I woke up in a hotel in Raleigh, North Carolina that Tuesday morning, and I saw a giant “StartupBus” decal on the charter coach outside my window, I had this realization that would have thrilled my younger self to no end: “Holy shit. I’m not just reporting a story about a hackathon, I have landed inside a real life competition show.”
The lesson you should take from Eric’s realization: if you think you wasted your time watching Survivor, The Amazing Race, or similar shows, guess what…you didn’t! A lot of the personal dynamics on those shows is pretty much like those on StartupBus. - Be prepared to pitch constantly. Eric observes: “To get the day started, each team sends one person to the front of the bus to practice their pitch over the intercom. This is something that happens a lot on StartupBus—people are practicing their pitches constantly.”
- Be prepared for surprise challenges and surprise obstacles. StartupBus borrows a big trick from reality TV shows: surprise obstacles. When the bus pulls into Charlotte, North Carolina, the New York team finds out that they’ll be pitching against two other teams — a bus that started in Akron, Ohio and another that started in…Tampa!
- Don’t limit yourself to just software, because there’s a chance that some team on another bus isn’t limiting itself to just software. “And the Ohio bus is impressive in its own way. It turns out they teamed up with some people from San Francisco, and they’re manufacturing physical products. So they have 3D printers and computer aided design software. The whole thing feels like that scene in “The Sandlot” when the other team shows up in their actual jerseys and matching converse sneakers, and all of a sudden you realize, ‘Oh… this is some real competition.’”
- Try not to fall into the trap of traditional gender dynamics. On one team, there’s a good news/bad news thing going on because they’re electing one of the women to be CEO; the bad news is that it’s a job that none of them particularly want, and a lot of it will be about reining in unruly behavior.
- Have a plan for managing conflict. On another team, a “that’s just who I am” kind of guy butts head against a woman on his team. This is a pretty big topic, and I’ll write more about it in a later post. Just know that you may have to manage conflict within the team.